Businesses must adapt quickly, innovate relentlessly, and ensure that their AI infrastructure can perform in ways that were once unimaginable.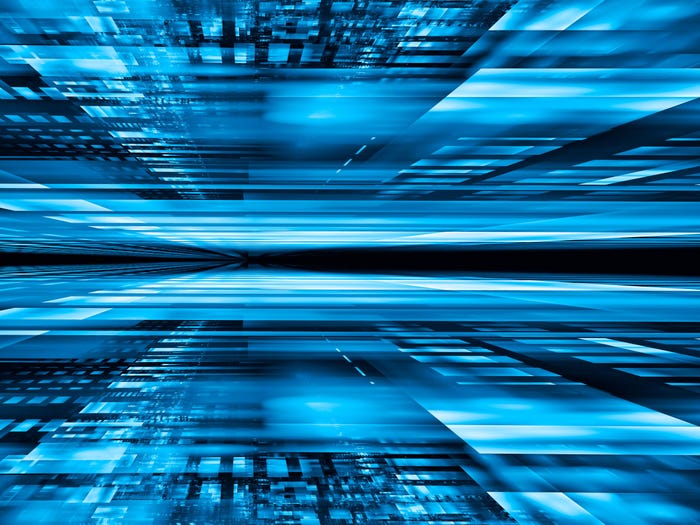
Network Management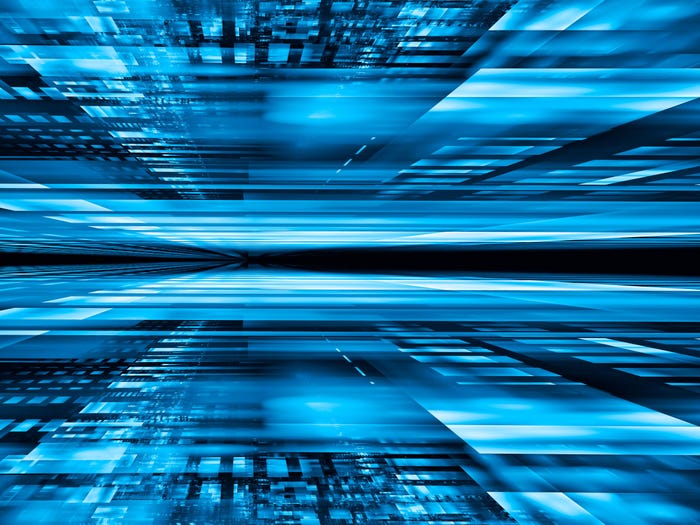
The AI Infrastructure Shift: Redefining Application DeliveryThe AI Infrastructure Shift: Redefining Application Delivery
To succeed today, businesses must adapt quickly, innovate relentlessly, and ensure that their AI infrastructure can perform in ways that were once unimaginable.
SUBSCRIBE TO OUR NEWSLETTER
Stay informed! Sign up to get expert advice and insight delivered direct to your inbox